Notifications are still experimental and may not work properly.
Please use notipy_osx==0.0.7
to use notifications.
Before creating notifications, ensure that your settings allow it. Go to System Preferences > Notifications
and make sure "Allow Notifications from Python" is enabled.
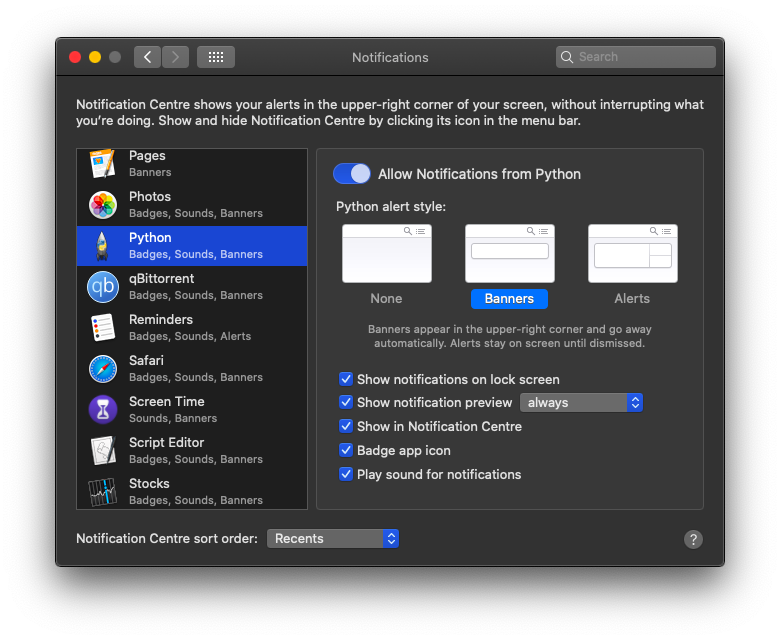
To create notifications, first import them like this:
from notipy_osx import notify
Parameters
title (string, required), subtitle (string), info_text (string), identity_image (relative image path), content_image (relative image path), delay (integer), sound (boolean)
title (string, required)
The title of the notification.
notify(title='Hello World')
# or
notify('Hello World')
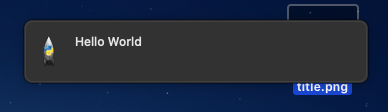
subtitle (string), and info_text (string)
The subtitle and informational text of the notification. Both are optional.
notify('Hello Notification', subtitle='The subtitle', info_text='The informational text')
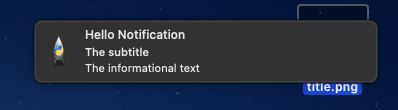
identity_image (path to image)
The icon to the left of the notification. This icon will come where the app icon is supposed to come.
In this example, I am setting the identity_image
to dot.png
, an image of a red dot.
notify('Hello Notification', identity_image='dot.png')
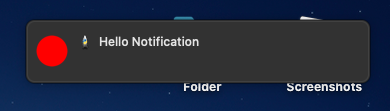
In Python scripts, the default icon is the "Python Rocket" (as seen in the above 2 images). The identity_image
will come in place of the "Python Rocket", but the "Python Rocket" will still be shown smaller, before the notification's title
text. The only way to change this default "Python Rocket" us to use py2app and create a Mac OS application. In this case, the default notification icon is the icon you give your application.
Check out this article for more information on py2app.
content_image (relative image path)
The icon to the right of the notification.
In this example, I am setting the content_image
to folder.png
, an image of a folder.
notify('Hello Notification', content_image='folder.png')
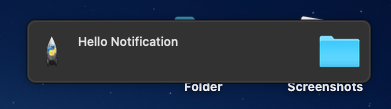
identity_image
and content_image
can be set at the same time:
notify('Hello Notification', identity_image='dot.png', content_image='folder.png')
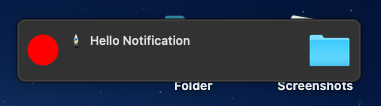
delay (integer)
The delay (in seconds) before sending the notification.
notify('Hello Notification', delay=10)
# the notification will be sent in 10 seconds
# even if the program has finished executing
It is possible for the program to finish executing before the notification is sent.
sound (boolean)
Whether to play the default Mac OS notification tone when the notification is send.
notify('Hello Notification', sound=True, delay=10)
# this notification will arrive in 10 seconds
# an play the default notification tone
Fallback
Notifications with PyObjC do not work on all devices. If it does not work, AppleScript is used as a fallback.
Unfortunately, only title
, subtitle
, and info_text
are supported. The icon will be set to the default AppleScript Editor icon.
If you wish to always AppleScript notifications, you may use it:
from notipy_osx import as_notify
as_notify(title='title', subtitle='sub', info_text='info')