To use create a dialog, import it first:
from notipy_osx import dialog_prompt
Parameters
text (string), buttons (list, strings), default_button (string), cancel_button (string), password, (boolean), icon (string).
text, string (required)
The text on the dialog.
dialog_prompt(text='Hello')
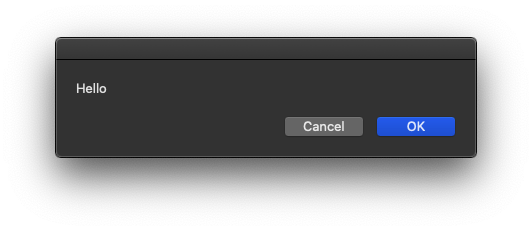
buttons, list of strings
The buttons to show below the text (or the user input). By default AppleScript puts the "Cancel" and "OK" buttons. These can be customized:
dialog_prompt(
text='Buttons example',
buttons=['Second', 'First']
)
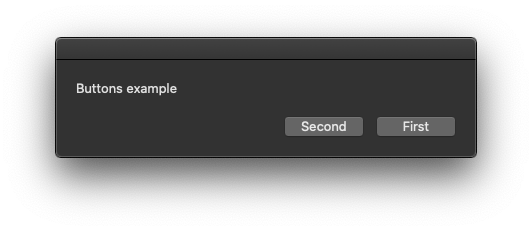
Maximum 3 buttons are allowed.
These buttons can be used to take user input.
default_button, string
The button that is highlighted blue and actived when the "return" key is pressed.
For example, we can set the button "First" as the default button:
dialog_prompt(
text='Buttons example',
buttons=['Second', 'First'],
default_button='First'
)
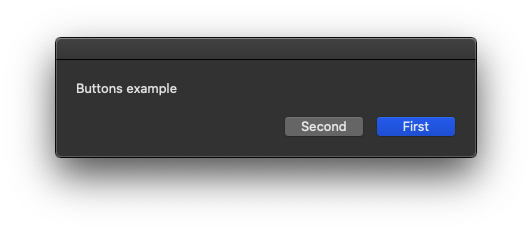
The string you put as the default_button
has to be in the buttons
list.
cancel_button, string
The button that "cancels" the dialog. When a cancel_button
is pressed, the dialog can be closed when the user hits the "escape" key.
If the parameter buttons
contains the string "Cancel", it is set as the cancel_button
by default. We can also explicitly set the cancel button.
dialog_prompt(
text='Buttons example',
buttons=['Second', 'First'],
default_button='First',
cancel_button='Second'
)
There are no visual changes, but the user can now use the "escape" key to close the dialog.
default_answer, string
The default text input. When default_answer
is set to an empty string, the text input is shown.
dialog_prompt(
text='Text input example',
default_answer=''
)
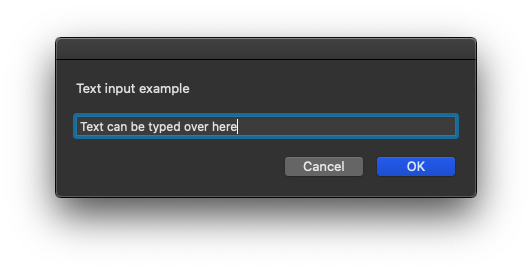
This text field can take user input.
password, boolean
Whether to use a password field (with hidden text). password
defaults to False
.
Note that setting password
to True
has no effect unless default_answer
is specified.
dialog_prompt(
text='Text input example',
default_answer='',
password=True
)
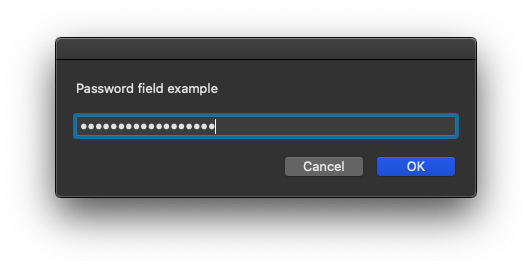
icon, string
The icon to show at the left side. By default, no icon is shown.
You may either provide a relative path to an icon, or use one of the three predefined icons from AppleScript (note
, caution
, or stop
)
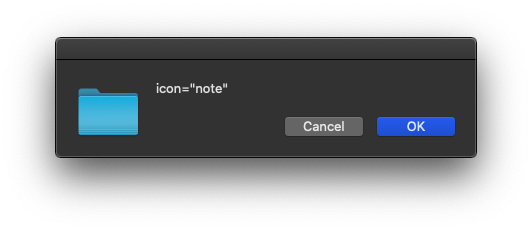
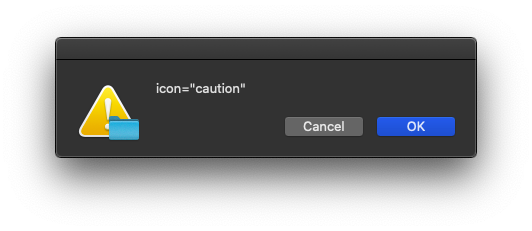
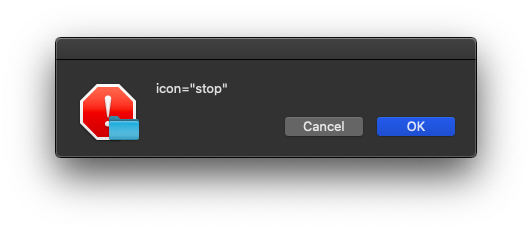
If you have an icon (Terminal.icns
, for example), you can specify the relative path:
prompt = dialog_prompt(
text='notipy_osx',
buttons=['Install', 'Star'],
default_button='Star',
# relative path
icon='icons/Terminal.icns'
)
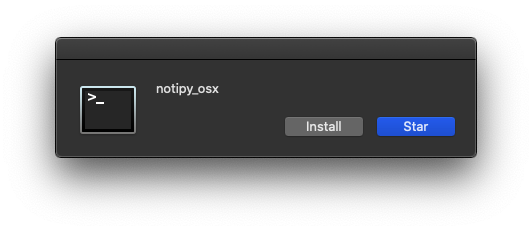
User input
the function dialog_prompt
returns a Result
object which contains the properties button_returned
and text_returned
.
Buttons
The return value of dialog_prompt
. will always contain button_returned
. For example,
answer = dialog_prompt(
text='Buttons example',
buttons=['Second', 'First']
)
print(answer.button_returned)
# prints either "Second" or "First"
With the above code, pressing the "return" key does not do anything. When a default_button
is set, the "return" key pressed that button automatically.
answer = dialog_prompt(
text='Buttons example',
buttons=['Second', 'First'],
default_button='First'
)
print(answer.button_returned)
# prints 'First' if the "return" key is pressed
Text
The return value of dialog_prompt
. will contain button_returned
if default_set
is specified. For example,
answer = dialog_prompt(
text='Buttons example',
buttons=['Second', 'First'],
default_button='First',
# specify default_answer for the text field to show
default_answer=''
)
print(answer.text_returned)
# prints the text you type in the text field
dialog_prompt
can return both text and button pressed.
answer = dialog_prompt(
text='Buttons example',
buttons=['Second', 'First'],
default_answer=''
)
print(answer.text_returned)
print(answer.button_returned)
The same applies with password
is set to True
.